使用产品:iserver 11.1.1a 操作系统:win11 x64
// 路径分析逻辑
const findPathProcess = (startPoint, endPoint) => {
const serviceUrl = 'http://localhost:8090/iserver/services/transportationAnalyst-CampusWorkspace-2/rest/networkanalyst/swpu_Network@Campus';
//创建最佳路径分析服务
const findPathService = new L.supermap.NetworkAnalystService(serviceUrl);
//创建最佳路径分析参数
const resultSetting = new L.supermap.TransportationAnalystResultSetting({
returnEdgeFeatures: true,
returnEdgeGeometry: true,
returnEdgeIDs: true,
returnNodeFeatures: true,
returnNodeGeometry: true,
returnNodeIDs: true,
returnPathGuides: true,
returnRoutes: true
});
const analystParameter = new L.supermap.TransportationAnalystParameter({
resultSetting: resultSetting,
weightFieldName: 'weight'
});
const findPathParameter = new L.supermap.FindPathParameters({
isAnalyzeById: false,
nodes: [L.point(...startPoint), L.point(...endPoint)],
parameter: analystParameter
});
console.log(findPathParameter)
const myIcon = L.icon({
iconUrl: '/Address.png',
iconSize: [20, 20]
});
findPathService.findPath(findPathParameter) // 调用findPathService对象的findPath方法,传入参数findPathParameter,用于查找路径
.then(function (serviceResult) { // 当findPath方法成功执行后,使用then方法来处理返回的结果
if (!serviceResult.result || !serviceResult.result.pathList || serviceResult.result.pathList.length === 0) { // 检查返回结果是否有效,即是否存在result对象、pathList数组以及pathList数组是否为空
console.error('No valid path found.'); // 在控制台输出错误信息,表示没有找到有效路径
alert('无法找到有效的路径'); // 弹出警告框,通知用户无法找到有效路径
return; // 结束当前函数执行
}
const result = serviceResult.result; // 将返回的结果赋值给变量result
currentPathLayer = L.layerGroup(); // 创建一个图层组currentPathLayer,用于存储路径相关的图层
result.pathList.forEach(function (path) { // 遍历pathList数组中的每一个路径
if (path.route) { // 如果路径包含route属性
console.log(path); // 打印整个路径对象,查看其结构
L.geoJSON(path.route).addTo(currentPathLayer); // 将路径的GeoJSON数据添加到图层组currentPathLayer
}
console.log(path.pathGuideItems)
if (path.pathGuideItems) { // 如果路径包含pathGuideItems属性
L.geoJSON(path.pathGuideItems, { // 将路径指南项目的GeoJSON数据添加到图层组currentPathLayer
pointToLayer: function (geoPoints, latlng) { // 定义如何将GeoJSON点要素转换为Leaflet图层
return L.marker(latlng, { icon: myIcon }).addTo(currentPathLayer); // 使用自定义图标myIcon在地图上创建标记,并添加到图层组currentPathLayer
},
filter: function (geoJsonFeature) {
return geoJsonFeature.geometry && geoJsonFeature.geometry.type === 'Point';
}
}).addTo(currentPathLayer); // 将处理后的GeoJSON数据添加到图层组currentPathLayer
}
});
if (currentPathLayer) { // 如果图层组currentPathLayer存在
currentPathLayer.addTo(map.value); // 将图层组currentPathLayer添加到地图上
}
})
.catch((error) => { // 如果findPath方法执行过程中出现错误,使用catch方法来捕获和处理错误
console.error('路径分析过程中发生错误:', error); // 在控制台输出错误信息
alert('路径分析失败,请检查网络分析服务配置'); // 弹出警告框,通知用户路径分析失败,并建议检查服务配置
});
};
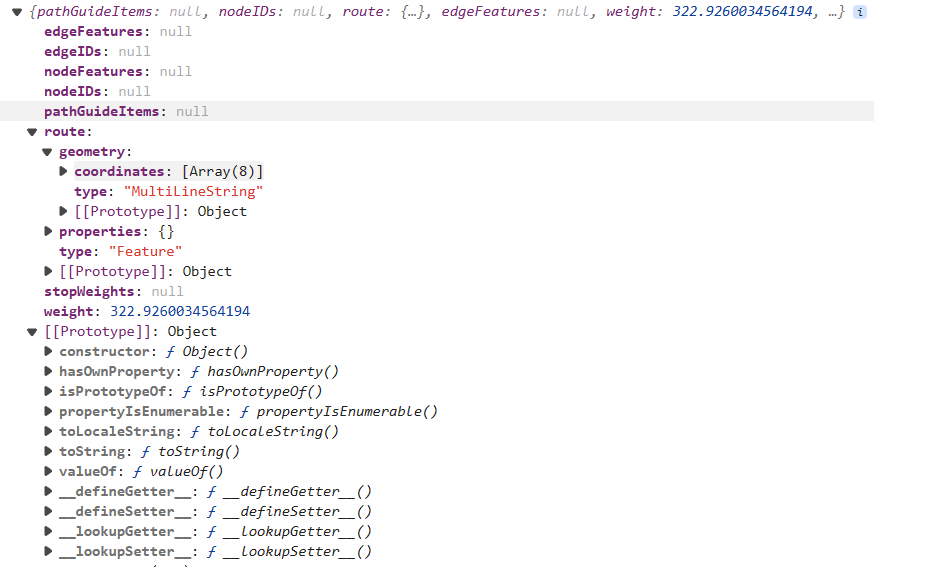